Common layouts
Learning objectives
- Knows how to create two- and three column layouts.
- Knows how to create grid-based layouts.
In addition to landing pages that often feature a Hero element and some additional information, the most common layout types are two- and three-column layouts. As an example, this course site has a two-column layout, although the left-hand side menu is implemented as a component that can be hidden. Let's look into building and using such layouts, starting with the following template that includes the TopBar
component but omits the Hero
component that we previously worked on.
---
import TopBar from "../components/TopBar.svelte";
---
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Hello world!</title>
</head>
<body>
<TopBar />
</body>
</html>
Two- and three column layouts
At the core of many common layouts is the idea that a page can be divided horizontally into equal width pieces, and these pieces can then be distributed over parts in the web application. In addition, they utilize the CSS flex property to allow growing and shrinking to fit the available space.
Tailwind comes with a basis utility class that can be used jointly with flex and flex direction to form layouts.
As an example, a two-column layout with a left hand side menu taking one sixth of the available space and the main area taking five sixths of the available space would be implemented as follows. In the following example, the left hand side has a light red background and the main area has a light green background that allows distinguishing them.
---
import TopBar from "../components/TopBar.svelte";
---
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Hello world!</title>
</head>
<body>
<TopBar />
<div class="flex flex-row">
<div class="bg-red-300 basis-1/6">left side</div>
<div class="bg-green-200 basis-5/6 pl-2">main</div>
</div>
</body>
</html>
The above example would produce a site that looks similar to the one shown in Figure 1.

As you adjust the width of the browser, you notice that the area available for the left side and the main area is adjusted accordingly. We could also add the container
and the mx-auto
property to create a situation where the size reserved for the two-column layout is constrained and the content is centered.
---
import TopBar from "../components/TopBar.svelte";
---
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Hello world!</title>
</head>
<body>
<TopBar />
<div class="container mx-auto flex flex-row">
<div class="bg-red-300 basis-1/6">left side</div>
<div class="bg-green-200 basis-5/6 pl-2">main</div>
</div>
</body>
</html>
The above page would look similar to the content shown in Figure 2.

Similarly, we could create a three column layout that has some content on both the left hand side and the right hand side, while keeping the main area in the middle. To reach this, the adjustment to the above example would be relatively small. We would simply add a new div that would be dedicated to the right side of the application, as shown below.
---
import TopBar from "../components/TopBar.svelte";
---
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Hello world!</title>
</head>
<body>
<TopBar />
<div class="container mx-auto flex flex-row">
<div class="bg-red-300 basis-1/6">left side</div>
<div class="bg-green-200 basis-4/6 pl-2">main</div>
<div class="bg-red-300 basis-1/6">right side</div>
</div>
</body>
</html>
The output of the above code is shown in Figure 3. As we observe, there are now three columns.

Grid-based layout
Another common approach for creating a layout is the use of the CSS grid property that can be used to declare a grid-based layout. As an example of a grid-based layout, see the main page of this course platform, where the cards representing courses are shown using a grid.
Tailwind provides utility classes grid
and grid-cols-*
that can be used to create a grid-based layout (see Grid Template Columns). The *
in grid-cols-*
is a number that describes the number of columns in the grid. As an example, if we would wish to have a grid with three columns, we would use the utility classes grid
and grid-cols-3
, as shown below.
---
import TopBar from "../components/TopBar.svelte";
---
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Hello world!</title>
</head>
<body>
<TopBar />
<div class="container mx-auto grid grid-cols-3">
<div class="bg-red-300">left side</div>
<div class="bg-green-200">main</div>
<div class="bg-red-300">right side</div>
</div>
</body>
</html>
The above example also has a container that is centered. The output of the above example similar to the output shown in Figure 4.

If we would add more elements to the user interface, the subsequent elements would be placed on a new row. In the following example, we have six elements in a grid layout with three columns, resulting in two rows. To distinguish the elements, we have also added the utility class gap-2
, which defines a 0.5rem
gap between the elements.
---
import TopBar from "../components/TopBar.svelte";
---
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Hello world!</title>
</head>
<body>
<TopBar />
<div class="container mx-auto grid grid-cols-3 gap-2">
<div class="bg-red-300">left side 1</div>
<div class="bg-green-200">main 2 </div>
<div class="bg-red-300">right side 3</div>
<div class="bg-red-300">left side 4</div>
<div class="bg-green-200">main 5</div>
<div class="bg-red-300">right side 6</div>
</div>
</body>
</html>
The output of the above example is shown in Figure 5.
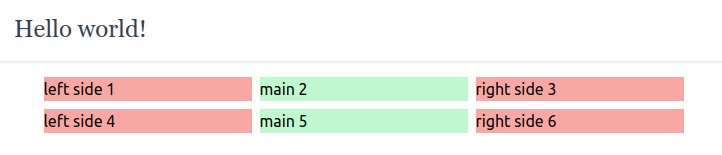
Grid layouts can also be used to achieve a layout similar to the column-based layout created with flex
earlier. For such a case, we would utilize col-span-*
in the elements, indicating the number of columns an individual element should occupy. As an example, the three column layout discussed previously could be reached by creating a grid with six columns, and having the first element take one column, the second element four columns, and the third element one column. In practice, as the default number of columns is one, we would have to define the constraint only to the second element. This would look as follows.
---
import TopBar from "../components/TopBar.svelte";
---
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Hello world!</title>
</head>
<body>
<TopBar />
<div class="container mx-auto grid grid-cols-6 gap-2">
<div class="bg-red-300">left side</div>
<div class="bg-green-200 col-span-4">main</div>
<div class="bg-red-300">right side</div>
</div>
</body>
</html>
The layout of the above web application would look similar to the one presented in Figure 6.
