Introduction to Svelte and tooling
Learning objectives
- You know what Svelte is.
- You know some tools that can be used to work with Svelte.
Introduction to Svelte
Svelte is a component-driven JavaScript framework for building client-side web applications. Components written in Svelte consist of JavaScript, HTML template, and styling (but may also omit the first and the last). As an example, the following is a Svelte component that is used to display the text Hello world!
in an h1
element.
<h1>Hello world!</h1>
In the above example, the component simply contains HTML. In the example below, the component contains both JavaScript and HTML. In the case of Svelte components, JavaScript is positioned at the top, which is followed by HTML. The component below shows the user the text "Hello world!" and a button. When the button is pressed, the text changes to "Hello Svelte!". Pressing the button again changes the text back to "Hello world!".
<script>
let content = "world";
const updateMessage = () => {
content = content === "world" ? "Svelte" : "world";
};
</script>
<h1>Hello {content}!</h1>
<button on:click={updateMessage}>Update!</button>
Similarly, the following is a Svelte component that demonstrates binding a variable into an input field. The following creates a component where the content that is typed into the input field is also reflected in a paragraph.
<script>
let typedContent = "";
</script>
<input bind:value={typedContent}>
<p>You typed {typedContent}.</p>
Svelte and React?
Svelte is a compiler-based framework, while React is a runtime-based library. Svelte converts components to optimized JavaScript at build time, while React uses a virtual DOM to update the browser in real time. Svelte also a simpler syntax, allowing a more direct utilization of prior JavaScript and HTML knowledge. At the same time, React has a larger developer community and a wider range of third-party libraries and tools.
Both are great options for client-side development. For an in-depth introduction to React, check out the Full Stack Open course.
Tooling
For working with Svelte locally, you need an editor (e.g. VSCode and the accompanying Svelte for VS Code plugin) and the Node.js package manager (npm) that comes with Node.js. We also strongly recommend nvm, which is a version manager for Node.js.
With npm
installed, you can create a Svelte project by running the command npm create svelte@latest
on the command line. Running the command asks to the package create-svelte@latest
, and then asks for the name of a folder into which the new project should be created. Once the folder has been entered, the program proceeds by asking for the type of project to create. Here, we'll choose "Skeleton project". This is followed by asking for type checking -- you can pick your preference; we'll choose "No". After this, the program asks whether we want to use a linter and whether we want to use a code formatter: of course we do! This is followed by a question on whether we want to use Playwright and Vitest for testing; pick an option that you prefer.
npm create svelte@latest
Need to install the following packages:
create-svelte@latest
Ok to proceed?
y
create-svelte version 2.3.2
Welcome to SvelteKit!
✔ Where should we create your project?
(leave blank to use current directory)
dab
✔ Which Svelte app template? ›
Skeleton project
✔ Add type checking with TypeScript? ›
No
✔ Add ESLint for code linting?
Yes
✔ Add Prettier for code formatting?
Yes
✔ Add Playwright for browser testing?
No
✔ Add Vitest for unit testing?
No
Your project is ready!
// ...
Next steps:
1: cd dab
2: npm install (or pnpm install, etc)
3: git init && git add -A && git commit -m "Initial commit" (optional)
4: npm run dev -- --open
To close the dev server, hit Ctrl-C
Now, when we run the commands (omitting the initialization of the git repository), we see the following.
cd dab
npm install
npm ERR! code EBADENGINE
npm ERR! engine Unsupported engine
npm ERR! engine Not compatible with your version of node/npm: @sveltejs/kit@1.2.10
npm ERR! notsup Not compatible with your version of node/npm: @sveltejs/kit@1.2.10
npm ERR! notsup Required: {"node":"^16.14 || >=18"}
npm ERR! notsup Actual: {"npm":"8.11.0","node":"v17.9.1"}
In our case, the Node version that we had installed is not supported. Fortunately, we have nvm
. Let's change the Node version to 16.15
.
nvm install 16.15
Downloading and installing node v16.15.1...
Downloading https://nodejs.org/dist/v16.15.1/node-v16.15.1-linux-x64.tar.xz...
################################################### 100,0%
Computing checksum with sha256sum
Checksums matched!
Now using node v16.15.1 (npm v8.11.0)
When we rerun the command npm install
, things look better.
npm install
added 144 packages, and audited 145 packages in 4s
30 packages are looking for funding
run `npm fund` for details
found 0 vulnerabilities
Now, we can finally launch the application.
npm run dev -- --open
Running the above command starts a development server and opens up a browser. The browser shows the text "Welcome to SvelteKit", as shown below in Figure 1.
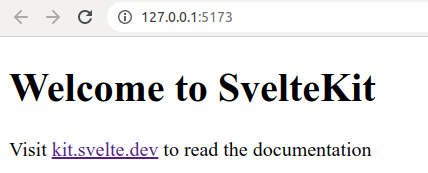
Pressing ctrl+C
in the terminal shuts down the development server. Let's not do it yet, though.
When we look into the folder into which the application is created, we see the following structure.
tree --dirsfirst
├── node_modules
| └── ...
├── src
│ ├── routes
│ │ └── +page.svelte
│ └── app.html
├── static
│ └── favicon.png
├── package.json
├── package-lock.json
├── README.md
├── svelte.config.js
└── vite.config.js
Let's create a folder called components
to the folder src
, and add a file called Hello.svelte
into it. Copy the following content to the file:
<script>
let message = 'Hello world';
</script>
<p>{message}!</p>
At this point, the folder structure should be as follows.
tree --dirsfirst
├── node_modules
| └── ...
├── src
│ ├── components
│ │ └── Hello.svelte
│ ├── routes
│ │ └── +page.svelte
│ └── app.html
├── static
│ └── favicon.png
├── package.json
├── package-lock.json
├── README.md
├── svelte.config.js
└── vite.config.js
Now, open up the file +page.svelte
in the folder routes
in the folder src
. The contents of the file are as follows.
<h1>Welcome to SvelteKit</h1>
<p>Visit <a href="https://kit.svelte.dev">kit.svelte.dev</a> to read the documentation</p>
Modify the file to match the following and save it.
<script>
import Hello from '../components/Hello.svelte';
</script>
<h1>Welcome to SvelteKit</h1>
<Hello />
Above, we load the component Hello
and add it to the component +page.svelte
. When we open up the browser again, the page looks now as follows.
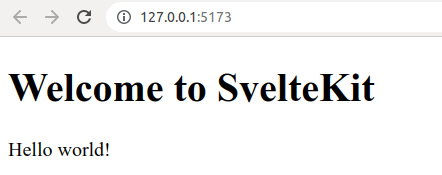
For a while, continue with minor modifications to Hello.svelte
to see how the changes reflect to what is being shown.
Svelte tutorial
Svelte has a really good online tutorial at https://svelte.dev/tutorial/basics. Check it out!