Introduction to Astro and tooling
Learning objectives
- You know what Astro is.
- You know some tools that can be used to work with Astro.
Introduction to Astro
Astro is a framework for building multi-page web applications. It has a component-based architecture, where pages, layouts etc are all components. Similar to Svelte, components written with Astro consist of JavaScript, HTML template, and styling, but you may also omit JavaScript and styling.
To start with, as an example, the following is an Astro component that is used to display the text Hello world!
in an h1
element (Déjà vu).
<h1>Hello world!</h1>
In an Astro application, routes are defined using components in the pages
folder. Linking is done with standard HTML anchor elements.
When considering JavaScript in Astro applications, JavaScript can be handled on the server and on the client. By default, Astro processes JavaScript on the server, generating static pages that are then served to the client.
Astro is a static site generator.
Astro is UI framework agnostic, which means that it supports a variety of user interface libraries and frameworks. In the present course, we'll with Astro and Svelte, but Astro works also with e.g. React and Preact, Vue, and Lit. When configured, Astro can simply import components from other UI libraries, even from multiple UI libraries at the same time would we choose to do so.
As Astro is a static site generator, it renders components on the server as static HTML. Also, by default, it attempts to not send unnecessary JavaScript to the client. This behavior can be controlled by directives that are used with components -- as an example, adding client:load
to a component definition describes that the JavaScript of the component should be handled on the client.
We'll dig into Astro in this chapter.
Tooling
To work with Astro, you need tooling. Tooling for Astro mostly matches the tooling for Svelte. In addition to npm
and an editor such as VSCode
, given that you use VSCode, you want to retrieve the Astro VS Code plugin.
A new Astro project is created using the npm create astro@latest
command. Running the command asks to the package astro@latest
, and then asks for the name of a folder into which the new project should be created. Once the folder has been entered, the program proceeds by asking for the type of project to create. Here, we'll choose "an empty project". This is followed by asking whether npm dependencies should be installed (choose "yes" for simplicity), which is followed by a question of whether a git repository should be initialized. We'll choose "no". After this, wishes for typescript type checking are prompted for -- we'll choose "Relaxed".
npm create astro@latest
Need to install the following packages:
create-astro@latest
Ok to proceed? (y)
y
╭─────╮ Houston:
│ ◠ ◡ ◠ Let's make the web a better place!
╰─────╯
astro v2.0.2 Launch sequence initiated.
✔ Where would you like to create your new project?
dab-astro
✔ How would you like to setup your new project? ›
an empty project
✔ Template copied!
✔ Would you like to install npm dependencies? (recommended)
yes
✔ Packages installed!
✔ Would you like to initialize a new git repository? (optional)
no
◼ Sounds good! You can come back and run git init later.
✔ How would you like to setup TypeScript? ›
Relaxed
✔ TypeScript settings applied!
next Liftoff confirmed. Explore your project!
Enter your project directory using cd ./dab-astro
Run npm run dev to start the dev server. CTRL+C to stop.
Add frameworks like react or tailwind using astro add.
Stuck? Join us at https://astro.build/chat
╭─────╮ Houston:
│ ◠ ◡ ◠ Good luck out there, astronaut!
╰─────╯
Now, when we run the commands outlined in the output -- cd dab-astro
and npm run dev
, we see the following.
cd dab-astro
npm run dev
> @example/minimal@0.0.1 dev
> astro dev
🚀 astro v2.0.2 started in 29ms
┃ Local http://localhost:3000/
┃ Network use --host to expose
Yay, the server is up and running. When we open the address localhost:3000
in a browser, the page looks as follows.
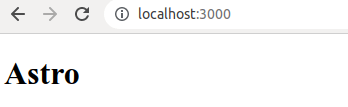
Pressing ctrl+C
in the terminal shuts down the development server. Let's not do it yet, though.
When we look into the folder into which the application is created, we see the following structure.
tree --dirsfirst
.
├── node_modules
| └── ...
├── public
│ └── favicon.svg
├── src
│ ├── pages
│ │ └── index.astro
│ └── env.d.ts
├── astro.config.mjs
├── package.json
├── package-lock.json
├── README.md
└── tsconfig.json
Let's create a folder called components
to the folder src
, and add a file called Hello.astro
into it. Copy the following content to the file:
<p>Hello world!</p>
At this point, the folder structure should be as follows.
tree --dirsfirst
.
├── node_modules
| └── ...
├── public
│ └── favicon.svg
├── src
│ ├── components
│ │ └── Hello.astro
│ ├── pages
│ │ └── index.astro
│ └── env.d.ts
├── astro.config.mjs
├── package.json
├── package-lock.json
├── README.md
└── tsconfig.json
Now, open up the file index.astro
in the folder pages
in the folder src
. The contents of the file are as follows.
---
---
<html lang="en">
<head>
<meta charset="utf-8" />
<link rel="icon" type="image/svg+xml" href="/favicon.svg" />
<meta name="viewport" content="width=device-width" />
<meta name="generator" content={Astro.generator} />
<title>Astro</title>
</head>
<body>
<h1>Astro</h1>
</body>
</html>
Modify the file to match the following and save it.
---
import Hello from "../components/Hello.astro";
---
<html lang="en">
<head>
<meta charset="utf-8" />
<link rel="icon" type="image/svg+xml" href="/favicon.svg" />
<meta name="viewport" content="width=device-width" />
<meta name="generator" content={Astro.generator} />
<title>Astro</title>
</head>
<body>
<h1>Astro</h1>
<Hello />
</body>
</html>
Above, we load the component Hello
from Hello.astro
and add it to index.astro
. When we open up the browser again, the page looks now as follows.
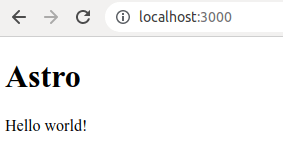
Try further minor modifications to Hello.astro
to see how the changes reflect to what is being shown.