Dynamic routes
Learning objectives
- You know how to create dynamic routes and know how to handle parameters from dynamic routes.
- You know that Astro can be configured to use server-side rendering instead of static site generation.
- You know how to enable static site generation for pages when Astro is configured to use server-side rendering.
Configuring server-side rendering
By default, Astro uses static site generation for creating the pages. To have dynamic routes that can be created and responded on the fly, we need to adjust Astro configuration to use server-side rendering.
Go to the file astro.config.mjs
in the root folder of your project, and add the property output
with the string value server
to the configuration object. After modification, the file should look as follows.
import { defineConfig } from 'astro/config';
// https://astro.build/config
export default defineConfig({
output: 'server',
});
Now, by default, Astro uses server-side rendering for generating the pages instead of static site generation.
For production use, a server-side runtime needs to be also configured for server-side rendering -- we'll look at this a bit later.
Static site generation for some pages
When Astro has been configured to use server-side rendering, pages that can be statically generated during build-time should include a line export const prerender = true;
at the top of the script of the page component. This tells Astro that those pages can be statically generated during build-time.
As an example, the index.astro
in the folder pages
could be adjusted as follows. Now, Astro will generate the corresponding page statically.
---
export const prerender = true;
import Layout from "../layouts/Layout.astro";
---
<Layout>
<p>Hello layout!</p>
</Layout>
Using dynamic routes
With server-side rendering enabled in Astro configuration, we can create dynamic routes. Dynamic routes still use file-based routing -- parameters are defined with a bracket notation and can be accessed through Astro.params
.
Create a folder called items
into the folder pages
, and create a file called [id].astro
into the folder items
. Copy the following contents to the file [id].astro
.
---
import Layout from "../../layouts/Layout.astro";
const id = Astro.params.id;
---
<Layout heading="Items">
<p>Here, we would show information about the item with id { id }!</p>
</Layout>
Now, when you access a path such as /items/3
, you will see a corresponding site. As an example, Figure 1 shows accessing the path /items/10430
.
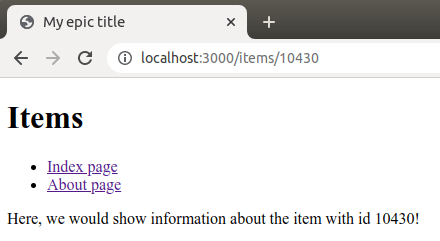
Dynamic routes and static site generation
Dynamic routes can also be combined with static site generation. In this case, the pages corresponding to the dynamic routes are generated during the build time. For this to work, the components corresponding to the dynamic routes need include the line export const prerender = true;
in the component definition, and to define a function getStaticPaths
that defines what paths will be generated.
As an example, when we adjust the [id].astro
in the folder items
as follows, only four pages will be generated.
---
export const prerender = true;
export const getStaticPaths = () => {
return [
{ params: { id: '70' } },
{ params: { id: '836' } },
{ params: { id: '4030' } },
{ params: { id: '5830' } },
];
}
import Layout from "../../layouts/Layout.astro";
const id = Astro.params.id;
---
<Layout heading="Items">
<p>Here, we would show information about the item with id { id }!</p>
</Layout>
The pages would be found at paths /items/70
, /items/836
, /items/4030
, and /items/5830
. As an example, Figure 2 shows the response to a client retrieving the page at path /items/70
.
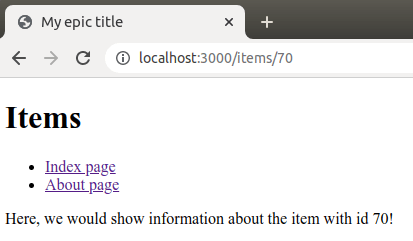
In this case, if we would query for a page at /items/1
, the response would be a page with the status code 404, i.e., Not Found. This is shown in Figure 3.
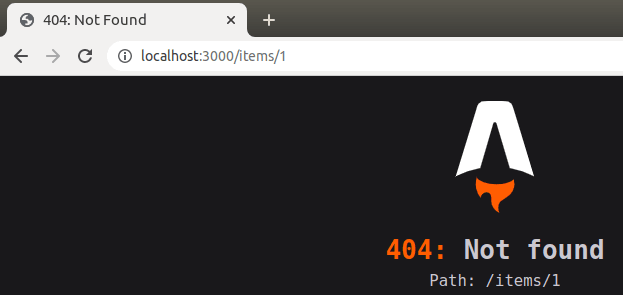