Tailwind and Astro
Learning objectives
- Knows what Tailwind CSS is.
- Knows how to add Tailwind CSS to an Astro application.
Tailwind CSS
Tailwind is a CSS framework that comes with a range of style classes that can be applied to HTML. The approach is rather similar to e.g. using Bootstrap, although it may feel a bit more verbose. When compared to using component libraries with styles (e.g. MUI), the approach is rather different as the developer has more freedom in styling.
The experiences that led to building Tailwind CSS are documented in a blog post by Adam Wathan, who is the creator of Tailwind CSS. Read the blog post at https://adamwathan.me/css-utility-classes-and-separation-of-concerns/ and come up with one multiple choice question on the blog article.
For writing the question, refer also to the notes on good questions.
Write the questions using the widget shown below.
Question not found or loading of the question is still in progress.
Once you have created the question, answer three or more peer-authored questions below. After each question, you are given a possibility to rate the question -- please, rate each question that you answer.
Question not found or loading of the question is still in progress.
Then, read the blog article Building a Scalable CSS Architecture, and come up with one more multiple choice question based on it. Write the questions using the widget shown below.
Question not found or loading of the question is still in progress.
Again, once you have created the question, answer three or more peer-authored questions below. After each question, you are given a possibility to rate the question -- please, rate each question that you answer.
Question not found or loading of the question is still in progress.
Adding Tailwind to Astro
We'll continue building straight on top of our application with Astro and Svelte. To add Tailwind CSS to the project, we run the command npx astro add tailwind
. The command asks whether we wish to install tailwind, whether Astro can generate a tailwind configuration, and whether Astro can adjust the Astro configuration. We'll answer yes to all.
npx astro add tailwind
✔ Resolving packages...
Astro will run the following command:
If you skip this step, you can always run it yourself later
╭─────────────────────────────────────────────────────────────────╮
│ npm install @astrojs/tailwind astro@^2.0.4 tailwindcss@^3.0.24 │
╰─────────────────────────────────────────────────────────────────╯
✔ Continue? …
yes
✔ Installing dependencies...
Astro will generate a minimal ./tailwind.config.cjs file.
✔ Continue? …
yes
Astro will make the following changes to your config file:
╭ astro.config.mjs ─────────────────────────────╮
│ import { defineConfig } from 'astro/config'; │
│ │
│ // https://astro.build/config │
│ import svelte from "@astrojs/svelte"; │
│ │
│ // https://astro.build/config │
│ import tailwind from "@astrojs/tailwind"; │
│ │
│ // https://astro.build/config │
│ export default defineConfig({ │
│ output: 'server', │
│ integrations: [svelte(), tailwind()] │
│ }); │
╰───────────────────────────────────────────────╯
✔ Continue? …
yes
success Added the following integration to your project:
- @astrojs/tailwind
Now, we have Tailwind CSS in our Astro project. In addition, you'll likely wish to add the Tailwind CSS IntelliSense into VSCode if you're using VSCode.
A first peek
With Tailwind included into our project, let's take a peek at what our application looks like. First, create an index.astro
to the pages
folder, and add the following contents to it.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Hello world!</title>
</head>
<body>
<span>Hello world!</span>
</body>
</html>
When you open up the page in a browser, you may notice that the page looks a bit different than before. As shown in Figure 1, the the font weight and the font are different. This stems from Tailwind including a base style into each page.
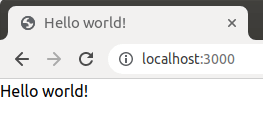
Let's continue by modifying the page so that it has a top bar. We'll use the nav
element as a starting point, and place the span
element into it.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Hello world!</title>
</head>
<body>
<nav>
<span>Hello world!</span>
</nav>
</body>
</html>
When you open up the above page in a browser, you'll notice that it has not changed at all and it looks similar to the previous version.
Tailwind CSS is an utility class library. When using Tailwind, the web applications are styled using the utility classes.
Top bars often come with some padding and margin. Padding is controlled using utility classes with the prefix p
, while margin is controlled using utility classes with the prefix m
. For example, with p-4
, we would use a padding of 1rem
in the element, while with mb-4
, we would use a margin of 1rem
at the bottom of the element. Let's add these to our nav
element.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Hello world!</title>
</head>
<body>
<nav class="p-4 mb-4">
<span>Hello world!</span>
</nav>
</body>
</html>
The output of the above adjustments is shown in Figure 2. The element nav
has now padding, which is shown from the way how the text Hello world!
is placed. There is also margin, although it is not observable from the image.
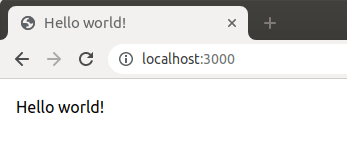
Let's next add a shadow under the navbar -- using the utility class shadow
, and adjust the font in the span
element. We adjust the size of the text and the color of the text using text-
-prefixed utilities, and change the font using font-serif
.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Hello world!</title>
</head>
<body>
<nav class="p-4 mb-4 shadow">
<span class="text-2xl text-gray-700 font-serif">Hello world!</span>
</nav>
</body>
</html>
The output of the above adjustments is shown in Figure 3. The element nav
has now also a shadow, and the font size and type have been changed.
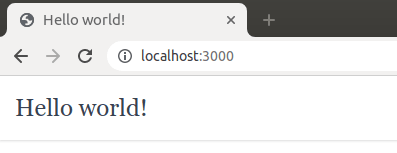
Let's continue by adding a container to the page. Containers are used to limit the width of a page and to center contents. In Tailwind, containers are defined using the container
class. The mx-auto
class is used to center the container in the window (not the contents of the container). We also add some padding for the container.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Hello world!</title>
</head>
<body>
<nav class="p-4 mb-4 shadow">
<span class="text-2xl text-gray-700 font-serif">Hello world!</span>
</nav>
<div class="container mx-auto p-2">
<p>Hello!</p>
</div>
</body>
</html>
The output of the above example is shown in Figure 4 below. There is a navigation bar at the top, and a container that would contain the body content of a page.
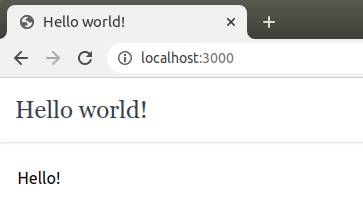