Eta Syntax
Learning objectives
- Knows how template values are used in Eta.
- Knows how to use JavaScript in Eta.
Let us dive deeper into Eta syntax which is used for inserting data from the server to view templates. Eta syntax can be divided into two parts, where one part is related to replacing template values with data from the server, and the other is related to JavaScript operations in files.
Note that in the following examples and assignments, we omit much of the HTML document content. In practice, when working with templates, the template files would contain the full HTML document -- at least until we learn to use partials and page layouts.
Template values
Previously, we briefly looked into using template values in our Eta documents. Template values are used in view templates for indicating positions into which data from the server is added. We already took a peek at this when adding a title and a count to a document. There are two ways of inserting data, which are as follows:
The format that starts with
<%=
, followed by a variable name with anit
-prefix, and a%>
, is used for inserting variable values to the document. In this format, possible HTML content in the variable is escaped, which means that HTML content from a variable is modified so that the content is not shown as HTML, but as text. In practice, this means that e.g. smaller than signs<
are represented with equivalent HTML entity tags, in this case<
. All variables passed to Eta from the server in have a prefixit
in Eta view templates, meaning that a variable calledname
from the server is added to an Eta document as<%= it.name %>
.The format that starts with
<%~
, followed by a variable name with anit
-prefix, and a%>
, is also used for inserting variable values to the document. In this format, however, possible HTML content in the variable is not escaped, which means that HTML content is shown as HTML. Similarly, to the previous, all variables from the server have a prefixit
, meaning that a variable calledname
from the server is added to an Eta document as<%~ it.name %>
.
Let's look at the difference of these two in practice. The following Eta document uses both formats for showing a variable. The %lt;
and >
are character entity references for the <
and >
signs. Let's assume that the following contents are in a file index.eta
in the views
folder.
<p>Trying out <%= it.variable %></p>
<%= it.variable %>
<p>Trying out <%- it.variable %></p>
<%~ it.variable %>
And the following are in our app.js
.
import { serve } from "https://deno.land/std@0.222.1/http/server.ts";
import { configure, renderFile } from "https://deno.land/x/eta@v2.2.0/mod.ts";
configure({
views: `${Deno.cwd()}/views/`,
});
const responseDetails = {
headers: { "Content-Type": "text/html;charset=UTF-8" },
};
const data = {
variable: '<marquee direction="up">Epic HTML!</marquee>',
};
const handleRequest = async (request) => {
return new Response(await renderFile("index.eta", data), responseDetails);
};
serve(handleRequest, { port: 7777 });
Now, when we launch the server with deno run --allow-net --allow-read app.js
, the application starts. When we make a request to the server using curl, we see the following output.
curl localhost:7777
<p>Trying out <%= it.variable %></p>
<marquee direction="up">Epic HTML!</marquee>
<p>Trying out <%- it.variable %></p>
<marquee direction="up">Epic HTML!</marquee>%
In practice, the first value has been escaped while the other has not. In a browser, the page will look as follows (except, the last line of text is continuously rotating from bottom to top).
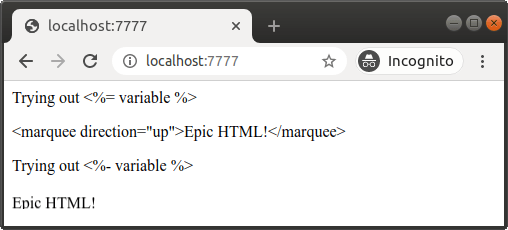
We will look into why escaping content from the server is important when discussing security concerns in web applications.
Question not found or loading of the question is still in progress.
JavaScript in Eta files
In addition to template values used for replacing HTML content with data, Eta supports using JavaScript in the documents. JavaScript can be added within a document using the format <% code %>
, where code
stands for code to be added.
Let us assume that the following code is within a file called index.eta
that resides within a folder called views
.
<%
for (let i = 0; i < 5; i++) {
console.log(i);
}
%>
Further, the following application is used send the contents created by the above view template to the user.
import { serve } from "https://deno.land/std@0.222.1/http/server.ts";
import { configure, renderFile } from "https://deno.land/x/eta@v2.2.0/mod.ts";
configure({
views: `${Deno.cwd()}/views/`,
});
const responseDetails = {
headers: { "Content-Type": "text/html;charset=UTF-8" },
};
const data = {
};
const handleRequest = async (request) => {
return new Response(await renderFile("index.eta", data), responseDetails);
};
serve(handleRequest, { port: 7777 });
When we launch the application and make a request to the server, we do not see any content in the response. This is due the content being processed on the server side.
curl localhost:7777
However, if we take a look at the server console, i.e. the console where the server application has been launched, we see the logged values.
deno run --allow-net --allow-read app.js
0
1
2
3
4
Mixing JavaScript and template values is straightforward. In the following example, we create an Eta file that shows a hello message for a particular name if such a variable name exists.
<% if (it.name) { %>
<h1>Hello, <%= it.name %></h1>
<% } %>
import { serve } from "https://deno.land/std@0.222.1/http/server.ts";
import { configure, renderFile } from "https://deno.land/x/eta@v2.2.0/mod.ts";
configure({
views: `${Deno.cwd()}/views/`,
});
const responseDetails = {
headers: { "Content-Type": "text/html;charset=UTF-8" },
};
const data = {
name: "Me!",
};
const handleRequest = async (request) => {
return new Response(await renderFile("index.eta", data), responseDetails);
};
serve(handleRequest, { port: 7777 });
curl localhost:7777
<h1>Hello, Me!</h1>
Let's look at the Eta file that uses both JavaScript and template values.
<% if (it.name) { %> <h1>Hello, <%= it.name %></h1>
<% } %>
At the beginning of the first line, the <%
-sign starts Eta JavaScript syntax (smaller than symbol, percentage symbol, and a whitespace). This is followed by an if
-statement, which checks for the existence of a variable called it.name
-- the it
is a prefix that notes that the variable comes from the server. The if
statement is opened with the curly bracket -- everything until the next closing curly bracket belongs within that if
statement. After the curly bracket, the Eta JavaScript syntax is closed with a %>
-sign (a whitespace, percentage symbol, and a greater than symbol).
The following line contains both HTML and a Eta template value.
<% if (it.name) { %>
<h1>Hello, <%= it.name %></h1><% } %>
The Eta template value <%= it.name %>
is replaced with the value of the variable name
-- in this case, when looking at the code on the server, Me!
. This, when combined with the HTML, creates the message that is shown as a response to the request, i.e. Hello Me!
.
On the last line, we close the if
-statement. The ending curly bracket matches the starting curly bracket, closing the block that contains the HTML heading and the template value.
<% if (it.name) { %>
<h1>Hello, <%= it.name %></h1>
<% } %>
Note that like any syntax, Eta is susceptible to syntax errors. If we, for example, leave out the last curly bracket, we will receive an empty response from the server.
<% if (it.name) { %>
<h1>Hello, <%= it.name %></h1>
<% %>
curl localhost:7777
curl: (52) Empty reply from server
deno run --allow-net --allow-read app.js
Eta Error: Loading file: /path-to-file/views/index.eta failed:
Bad template syntax
Unexpected token ')'
====================
// ... further details details
In the above example, the error indicates that the template syntax was erronous. From this, we can suspect that the fault lies in the Eta file, which we would look into for possible issues.