Svelte and npm
Learning objectives
- You know what Svelte is and you know what npm is.
- You know how to create a new Svelte application.
Svelte is a framework for building client-side web applications. In this chapter, we delve to the basics of Svelte, which we then build on top of in the following chapters.
Svelte uses Node Package Manager (npm) for managing its dependencies and for running a new project wizard. In addition, npm is used as the entrypoint for running a development server and building the project.
Installing npm
To get started with Svelte, you need to install npm. The recommended approach for installing npm (and Node.js) is installing a Node Version Manager (nvm), which allows you to install multiple versions of Node.js and npm. You can install nvm on Windows, macOS, and Linux -- the installation instructions are available at https://github.com/nvm-sh/nvm.
Alternatively, you may also use the Node.js downloads site at https://nodejs.org/en/download and download and install the latest LTS version of Node.js and npm.
Once you have installed npm, you may proceed.
Creating a new Svelte project
To create a new Svelte project, we run the command npm create svelte@latest
, which is followed by the name of the folder that we wish to use for creating the project. Below, we use the folder name svelte-ui
.
Note that although the version of Svelte 5 is still in preview mode, these materials have been written to use Svelte 5. Some functionalities that we use are not present in the older versions (and some functionality might change upon the 5.0 version release).
The command launches a wizard, which is used to configure the project. The wizard first asks to install the create-svelte
package that is used for creating the project. We allow this. Then, the wizard asks us to select a template for the project. We select the Skeleton project
template. The wizard also asks us if we wish to add type checking with TypeScript. We select No
. Finally, the wizard asks us to select additional options -- we wish to try out Svelte 5 preview and select that. The wizard then creates the project.
The interaction is as follows.
npm create svelte@latest svelte-ui
create-svelte version 6.1.1
┌ Welcome to SvelteKit!
│
◇ Which Svelte app template?
│ Skeleton project
│
◇ Add type checking with TypeScript?
│ No
│
◇ Select additional options (use arrow keys/space bar)
│ Try out Svelte 5 preview (unstable!)
│
└ Your project is ready!
The wizard also outputs the following instructions (and a bit more).
Next steps:
1: cd svelte-ui
2: npm install
3: git init && git add -A && git commit -m "Initial commit" (optional)
4: npm run dev -- --open
To close the dev server, hit Ctrl-C
Let's follow the instructions, and see what we have got. First, we change the directory to the project folder, and list the files in the folder.
cd svelte-ui
tree --dirsfirst
.
├── src
│ ├── lib
│ │ └── index.js
│ ├── routes
│ │ └── +page.svelte
│ └── app.html
├── static
│ └── favicon.png
├── package.json
├── README.md
├── svelte.config.js
└── vite.config.js
4 directories, 8 files
For the present version of the materials, we are using the following contents for the package.json
file
{
"name": "svelte-ui",
"version": "0.0.1",
"private": true,
"scripts": {
"dev": "vite dev",
"build": "vite build",
"preview": "vite preview"
},
"devDependencies": {
"@sveltejs/adapter-auto": "3.2.0",
"@sveltejs/kit": "2.5.6",
"@sveltejs/vite-plugin-svelte": "3.1.0",
"svelte": "5.0.0-next.107",
"vite": "5.2.9"
},
"type": "module"
}
Then, we run the npm install
command in the project folder, which downloads the dependencies into the project. The dependencies are downloaded to a folder called node_modules
, which is created into the project. The command might take a while to run.
npm install
added 53 packages, and audited 54 packages in 15s
4 packages are looking for funding
run `npm fund` for details
found 0 vulnerabilities
Once the npm install
command has been run, skip the part on initiating a git repository. Instead, we start the development server by running the command npm run dev -- --open
. The command also opens up a browser.
Running the command produces the following output.
npm run dev -- --open
> svelte-ui@0.0.1 dev
> vite dev --open
[...] ...notifications
VITE v5.2.9 ready in 478 ms
➜ Local: http://localhost:5173/
➜ Network: use --host to expose
➜ press h to show help
The browser window that is opened shows the following page.
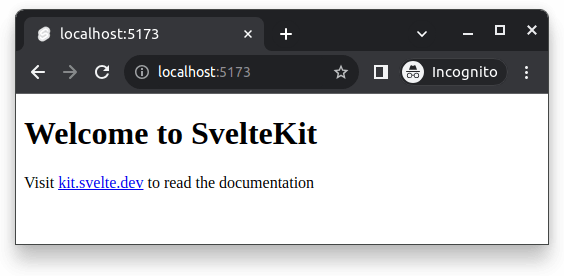
The above window contains the word "SvelteKit". SvelteKit is a scaffolding for building web applications with Svelte -- in this course, we'll mostly focus on Svelte, omitting much of the potential of SvelteKit.
The contents of the page come from the file +page.svelte
that is in the folder src/routes
. The file contains the following code.
<h1>Welcome to SvelteKit</h1>
<p>Visit <a href="https://kit.svelte.dev">kit.svelte.dev</a> to read the documentation</p>
If you change the contents of the file and save the file, you should notice that the contents shown in the browser also change. This is because the development server is running in the background, and it automatically updates the contents of the browser when the contents of the file change. Once you've tried this out, revert the contents of the file back to the original.