Component Properties
Learning objectives
- You know how to pass information to a Svelte component.
When web applications are composed of components, one of the recurring need is to pass information to the components. One way to achieve this is through the use of properties, which are values that are passed to a component when it is used.
Let's create a new component called QuestionAndAnswer.svelte
that will display a question and an answer. Create the file and store it to the components
folder under lib
. After the change, the folder structure should be as follows.
.
├── node_modules
│ └── (plenty of stuff)
├── src
│ ├── lib
│ │ ├── components
│ │ │ ├── Hello.svelte
│ │ │ └── QuestionAndAnswer.svelte│ │ └── index.js
│ ├── routes
│ │ └── +page.svelte
│ └── app.html
├── static
│ └── favicon.png
├── package.json
├── README.md
├── svelte.config.js
└── vite.config.js
Declaring component properties
In Svelte, component properties are declared in the script area of a template. They are declared using let { name } = $props();
, where name
is the name of the property. If there are multiple properties, they are separated from each others using commas.
Let's add two properties to the QuestionAndAnswer.svelte
component: question
and answer
. With the properties declared, the component should look as follows.
<script>
let { question, answer } = $props();
</script>
By default, the properties do not have values. Let's add default values to the properties -- default values are shown if values are not passed for the properties. Modify the above to match the following.
<script>
let { question = "What is the meaning of life?", answer = 42 } = $props();
</script>
If you wish to not use any default values, you could also explicitly define the default values as
undefined
.
Using properties in HTML
Properties are used in the HTML template area of a component. They are used by enclosing them in curly braces. Let's use the properties in the QuestionAndAnswer.svelte
component as a part of a sentence If the question is "...", then the answer is "..."
. Adding the sentence to the component, with the properties used, should look as follows.
<script>
let { question = "What is the meaning of life?", answer = 42 } = $props();
</script>
<p>If the question is "{question}", then the answer is "{answer}".</p>
{} -- JavaScript Expressions
Curly brackets in the template area denote a JavaScript expression. Writing {question}
evaluates the question
variable, which results to the {question}
being replaced by the value in question
. As curly brackets denote JavaScript expressions, you can write any sort of JavaScript expression within the curly bracket -- as an example, {question[0]}
would display the first character of the question.
Importing a component with properties
To use the component, we need to again import it. Let's modify the src/routes/+page.svelte
file to import the QuestionAndAnswer
component. The import statement should be added to the script area of the file, as follows.
<script>
import Hello from '$lib/components/Hello.svelte';
import QuestionAndAnswer from '$lib/components/QuestionAndAnswer.svelte';
</script>
<Hello />
<p>Visit <a href="https://kit.svelte.dev">kit.svelte.dev</a> to read the documentation</p>
Above, we are not yet using the component, even though it is imported. Let's add a QuestionAndAnswer
component to the HTML template area, after the Hello
component.
<script>
import Hello from '$lib/components/Hello.svelte';
import QuestionAndAnswer from '$lib/components/QuestionAndAnswer.svelte';
</script>
<Hello />
<QuestionAndAnswer />
<p>Visit <a href="https://kit.svelte.dev">kit.svelte.dev</a> to read the documentation</p>
When we run the application (if it is not yet running) with the command npm run dev -- --open
, the browser page opens up and we are shown a page that looks as follows.
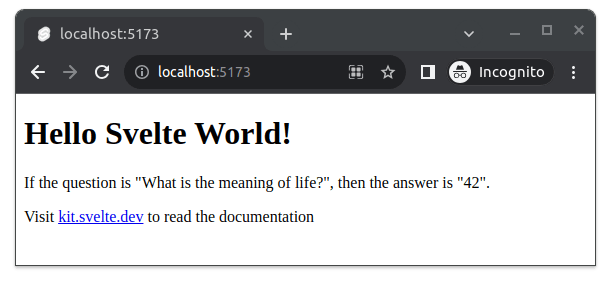
As we observe from the above image, we see the text If the question is "What is the meaning of life?", then the answer is "42".
as a part of the page. This is the text that we added to the QuestionAndAnswer
component -- as we did not pass any values for the properties, the default values are used.
Assigning property values
Let's modify the src/routes/+page.svelte
file to pass a value for the question
property of the QuestionAndAnswer
component. Passing values for components is done in a similar fashion as defining attributes for HTML elements.
That is, to pass a property value to a component, we add an attribute to the component, using the property name as the attribute name and the passed value as the attribute value. In the following, we pass the question "How much is 38+4?" to to the QuestionAndAnswer
component.
<script>
import Hello from '$lib/components/Hello.svelte';
import QuestionAndAnswer from '$lib/components/QuestionAndAnswer.svelte';
</script>
<Hello />
<QuestionAndAnswer question="How much is 38+4?" />
<p>Visit <a href="https://kit.svelte.dev">kit.svelte.dev</a> to read the documentation</p>
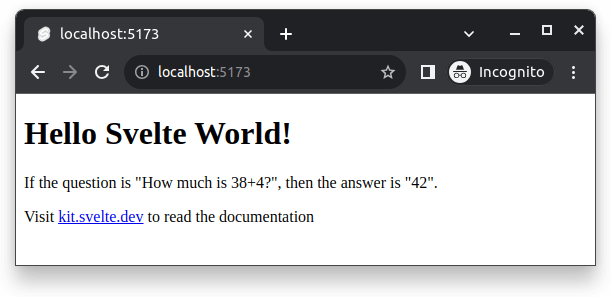
Similarly, we can pass a value for the answer
property. Let's modify the src/routes/+page.svelte
file to pass values to both question
and answer
.
<script>
import Hello from '$lib/components/Hello.svelte';
import QuestionAndAnswer from '$lib/components/QuestionAndAnswer.svelte';
</script>
<Hello />
<QuestionAndAnswer question="What's next?" answer="Wait and see" />
<p>Visit <a href="https://kit.svelte.dev">kit.svelte.dev</a> to read the documentation</p>
With the above change, the contents of the page are now as follows.
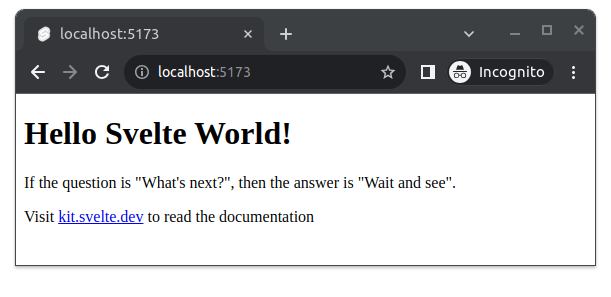
Spread and property values
In addition to passing individual property values, we can also pass multiple property values at once. This is done using the spread operator ...
that is used with JavaScript objects. Let's modify the src/routes/+page.svelte
to include a JavaScript object in the script area as follows.
<script>
import Hello from "$lib/components/Hello.svelte";
import QuestionAndAnswer from "$lib/components/QuestionAndAnswer.svelte";
let qa = {
question: "Can this work?",
answer: "Yes it can!",
};
</script>
<!-- template etc -->
Now, instead of passing individual property values using arguments, we can pass the object qa
to the QuestionAndAnswer
component. This is done by using the spread operator ...
with the object name, in curly brackets, as follows.
<QuestionAndAnswer {...qa} />
Jointly, the src/routes/+page.svelte
file should look as follows.
<script>
import Hello from "$lib/components/Hello.svelte";
import QuestionAndAnswer from "$lib/components/QuestionAndAnswer.svelte";
let qa = {
question: "Can this work?",
answer: "Yes it can!",
};
</script>
<Hello />
<QuestionAndAnswer {...qa} />
<p>
Visit <a href="https://kit.svelte.dev">kit.svelte.dev</a> to read the documentation
</p>
With the above change, the contents of the page are now as follows.
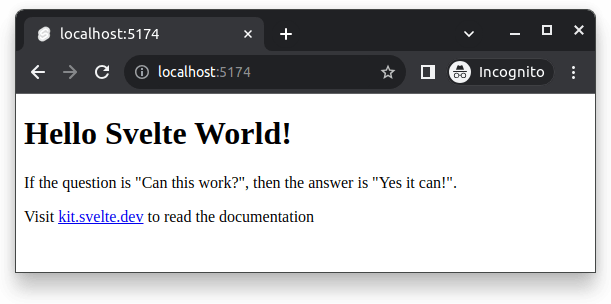
The spread operator will become quite handy when we learn more about working APIs, lists of components, and so on.