Layout File and Pages
Learning objectives
- You know how to create a layout in Svelte.
- You know how to create multiple pages in Svelte.
- You know how to navigate between pages in Svelte.
- You know how to define page-specific titles.
What we're looking into here is related to SvelteKit. SvelteKit is a framework that is built on top of Svelte, which makes creating web applications slightly easier. When using the command
npm create svelte@latest
, we are actually creating a SvelteKit project.
Defining a layout
The layout of an application is defined in a file called +layout.svelte
file that is placed in the folder src/routes/
of the application. The layout file is a regular Svelte component that can contain other Svelte components. The layout file is used to define the common layout of the application, including a header, a footer, and a possible navigation bar. In addition to the common parts, the layout file should also contain a <slot />
element that is used to render the actual content of the page -- that is, the content from the +page.svelte
.
Let's create a layout file for our application. Create a file called +layout.svelte
in the folder src/routes/
, and place the following contents to the file.
<header>
<h1>My application</h1>
<nav>
<a href="/">Home</a>
</nav>
</header>
<div>
<slot />
</div>
<footer>
<p>My application is cool.</p>
</footer>
Next, modify the +page.svelte
so that the content of the file is as follows.
<p>Hello world!</p>
Now, when you run the application and open it up in a browser, the application looks as follows.
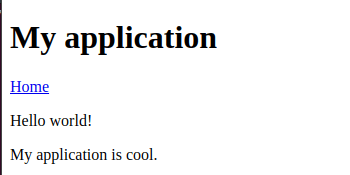
Creating pages
So far, our Svelte applications have had a single page. This is absolutely ok -- many applications have only a single page. However, it is also possible to have multiple pages. When working with Svelte, each page corresponds to a folder in src/routes/
, where the name of the folder is used to define the path of the page. In addition, each folder must have a +page.svelte
file that defines the content of the page.
To demonstrate this, let's create two new folders to src/routes/
. The first folder is about
, and the second folder is contact
. In both folders, create a file called +page.svelte
. For the about
folder, use the following content for +page.svelte
.
<p>This is my about page!</p>
And, for the contact
folder, use the following content for the +page.svelte
.
<p>You can contact me here.</p>
At this point, the contents of the src/routes
folder (and its subfolders) should be as follows.
tree --dirsfirst
.
├── about
│ └── +page.svelte
├── contact
│ └── +page.svelte
├── +layout.svelte
└── +page.svelte
2 directories, 4 files
Now, when you run the application and open it up in a browser, you can modify the address to see the pages. As an example, the about page looks as follows.
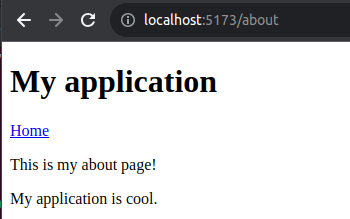
As you can see, the layout is used for all the pages of the application.
Navigating between pages
When working with multiple pages, it is important to be able to navigate between the pages. To achieve this, we use vanilla HTML links -- there's already a nice location for the navigation in our layout file. Let's add a link to the about page to the navigation bar. Modify the +layout.svelte
file so that the navigation bar looks as follows.
<header>
<h1>My application</h1>
<nav>
<a href="/">Home</a>
<a href="/about">About</a>
<a href="/contact">Contact</a>
</nav>
</header>
<div>
<slot />
</div>
<footer>
<p>My application is cool.</p>
</footer>
Now, as we've modified the layout, all of the pages also have links.
Page-specific titles
When working with multiple pages, it is often useful to have a page-specific title for each page. This can be achieved by using the title
attribute of the svelte:head
element. The svelte:head
element is used to define the contents of the <head>
element of the page. Let's add a page-specific title to the about page. Modify the +page.svelte
file in the about
folder so that the contents of the file are as follows.
<svelte:head>
<title>About</title>
</svelte:head>
Now, when you visit the page, you notice that the title contains the page-specific title.
The underlying reason why this works is that SvelteKit injects the contents of
svelte:head
to the location marked with%sveltekit.head%
inapp.html
insrc
. If you take a peek atapp.html
, the file looks as follows.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8" />
<link rel="icon" href="%sveltekit.assets%/favicon.png" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
%sveltekit.head%
</head>
<body data-sveltekit-preload-data="hover">
<div style="display: contents">%sveltekit.body%</div>
</body>
</html>
Advanced routing
For advanced routing, check out SvelteKit documentation at https://kit.svelte.dev/docs/advanced-routing.