TailwindCSS and DaisyUI
Learning objectives
- You know what TailwindCSS and DaisyUI are.
- You know how to use DaisyUI (and TailwindCSS) in your project.
Nowadays, it is becoming increasingly rare to create styles from scratch, and most developers rely on CSS libraries and frameworks. A CSS framework is a collection of CSS files that contain predefined CSS rules and styling for common elements. There are many CSS frameworks available -- one of the more popular ones is TailwindCSS, which provides utility classes for styling elements. CSS frameworks are often used jointly with component libraries, which are collections of components (or styles that create components) that are used when building the website. One such component library is DaisyUI, which builds on top of TailwindCSS.
Here, we look into taking TailwindCSS and DaisyUI into use in our Svelte project.
Installing TailwindCSS and DaisyUI
To install TailwindCSS and DaisyUI to the Svelte project, first navigate to the project folder. The contents of the folder should be similar to the following.
tree --dirsfirst -L 1
.
├── node_modules
├── src
├ ── static
├── package.json
├── package-lock.json
├── README.md
├── svelte.config.js
└── vite.config.js
In that folder, run the command npm install -D tailwindcss@latest @tailwindcss/typography postcss@latest autoprefixer@latest daisyui@latest
. This installs the latest versions of TailwindCSS, PostCSS, Autoprefixer and DaisyUI, as well as a typography plugin for TailwindCSS. The -D
flag indicates that the packages are installed as development dependencies, which means that they are not included in the production build of the project.
npm install -D tailwindcss@latest @tailwindcss/typography postcss@latest autoprefixer@latest daisyui@latest
The command outputs a handful of warnings, but do not mind. Next, run the command npx tailwindcss init
to create a tailwind.config.js
file in the project folder. This file is used to configure TailwindCSS.
npx tailwindcss init
At this point, the folder has a new file called tailwind.config.js
-- the folder contents are as follows.
tree --dirsfirst -L 1
.
├── node_modules
├── src
├── static
├── package.json
├── package-lock.json
├── README.md
├── svelte.config.js
├── tailwind.config.js
└── vite.config.js
The contents of tailwind.config.js
are as follows (or similar to the following).
cat tailwind.config.js
/** @type {import('tailwindcss').Config} */
export default {
content: [],
theme: {
extend: {},
},
plugins: [],
}
Configuring TailwindCSS and DaisyUI
First, modify the tailwind.config.js
file to match the following. The content
property is used to tell TailwindCSS to look for content from all .js
and .svelte
files in the src
folder, and the plugins
property is used to tell TailwindCSS to use the typography plugin and DaisyUI.
export default {
content: ["./src/**/*.{js,svelte}"],
plugins: [require("@tailwindcss/typography"), require("daisyui")],
}
Next, create a postcss.config.js
file in the project folder. This file is used to configure PostCSS, which is used to process CSS by TailwindCSS and consequently by DaisyUI. The contents of the file postcss.config.js
should be as follows.
export default {
plugins: {
tailwindcss: {},
autoprefixer: {},
},
}
Adding styles to project
Next, add a file app.css
to the src
folder, and copy the following Tailwind directives to the file.
@tailwind base;
@tailwind components;
@tailwind utilities;
The above directives are used to tell TailwindCSS to include the base styles, components and utilities to the application.
Then, modify the +layout.svelte
in the src/routes
folder to include the app.css
file. The file could look, for example, as follows.
<script>
import "../app.css";
</script>
<header>
<h1>My application</h1>
<nav>
<a href="/">Home</a>
<a href="/about">About</a>
<a href="/contact">Contact</a>
</nav>
</header>
<div>
<slot />
</div>
<footer>
<p>My application is cool.</p>
</footer>
Finally, modify the the +page.html
in the src/routes
to match the following.
<svelte:head>
<title>My application</title>
</svelte:head>
<p>Hello world!</p>
Now, when you open up the application in the browser, you should see something similar to the following.
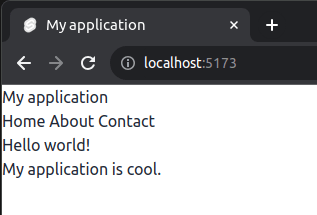
The application has been styled with the default styles of TailwindCSS, but the styles are not yet what we want. Let's look into fixing that next.
DaisyUI logged multiple times
The server that we use to launch Svelte for development might log DaisyUI to the console multiple times. This is a known issue, and it is not a problem.