HTML and Document Structure
Learning objectives
- You know what HTML is and what it is used for.
- You know how HTML documents are structured.
So far, we have learned the very basics of core server-side web application functionality, including working with routes (paths and methods and mapping them into functions), and returning responses that depend on these. We've also briefly looked into storing data on the server. Our applications have not had an user interface, as the applications have not used response formats that the browser would meaningfully interpret and display. This is where Hypertext markup language (HTML) comes into play.
HTML is a markup language that is used for defining the structure and content of web pages. Browsers interpret the HTML, and create the web application user interface based on it.
HTML in Browser
Copy the following content to a file called app.js
and run the file using Deno.
import { Hono } from "https://deno.land/x/hono@v3.12.11/mod.ts";
const app = new Hono();
app.get("/", async (c) => {
return c.html(`<!DOCTYPE html>
<html>
<head>
<title>Title</title>
</head>
<body>
<h1>Magic!</h1>
<p>Now, we will familiarize ourselves with HTML.</p>
</body>
</html>`);
});
Deno.serve(app.fetch);
When you open up the browser and navigate to http://localhost:8000
, you will see the something like the following.
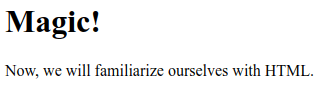
The above example application served HTML using Hono. The application works almost the same way as our previous applications. However, instead of using the method text
of Hono's context, we use the method html
. The method html
adds information -- a content-type header -- to the response, incidating that the response should be interpreted as HTML. When the browser receives such a response, it interprets it, and creates a user interface based on it.
Even though the content is interpreted by the browser, on the command line, we can see the raw HTML content. This is shown below.
curl localhost:8000
<!DOCTYPE html>
<html>
<head>
<title>Title</title>
</head>
<body>
<h1>Magic!</h1>
<p>Now, we will familiarize ourselves with HTML.</p>
</body>
</html>
Structure of a HTML Document
Each HTML document consists of a header row, elements, and text. The header row <!DOCTYPE html>
tells that the document is a HTML document. After the header row, an HTML document has an html
-element, which contains the contents of the HTML page. The contents are divided into header (head
-element) and body (body
-element). An element is defined using a starting tag that consists of a smaller than character <
and a greater than character >
, where the name of the element is given between the smaller than and greater than characters (e.g. <html>
, <head>
, <body>
).
Each element can contain an arbitrary number of other elements or text. Each element ends with an ending tag, which is similar to the starting tag but has a slash (e.g. </html>
). The exception is void elements with no elements or text within, which are written with a slash at the end (e.g. <br/>
, which indicates a line change).
Each element opens up an area that must be closed. As an example, the
html
-element (<html>
) that comes after the header row starts an HTML document. The page contents -- both the header and the body -- go into thehtml
element. Thehtml
element is closed at the end of the document (/html>
). Other elements follow the same principle -- an element is opened by writing<element>
and closed by writing</element>
.
A header -- the head
element -- contains elements which are not visible on the page, but may influence the behavior of the browser. As an example, the title
-element within the header is used to define a page title, which will be shown on the browser tab. The body
element on the other hand contains information that can be visible in the browser.
As an example, let us look at the following HTML document. The document has a heading that defines a title. The text "Title" would be shown in a browser tab. The document also has a body with two elements. The first element is a main heading (h1
), and the second element is a text paragraph (p
). Both of these contain text.
<!DOCTYPE html>
<html>
<head>
<title>Title</title>
</head>
<body>
<h1>Welcome!</h1>
<p>Now, we will familiarize ourselves with HTML.</p>
</body>
</html>
When viewed in a browser, the above document looks as follows.
HTML Document as a Tree
Each HTML document can be thought of a upside-down tree, where the root is the html
-element. The html
-element has two branches, head
and body
. Both of these can branch further. Below, you can see the previously shown HTML document and a tree-like representation that corresponds to it.
<!DOCTYPE html>
<html>
<head>
<title>Title</title>
</head>
<body>
<h1>Welcome!</h1>
<p>Now, we will familiarize ourselves with HTML.</p>
</body>
</html>
The body has two elements (h1
and p
), both of which contain text. Both the heading and the body can contain branches -- the following example uses a section
-element to represent a part of the page. The element section
contains two paragraphs (represented with p
-element) of text.
<html>
<head>
<title>Title</title>
</head>
<body>
<h1>Welcome!</h1>
<section>
<p>First paragraph.</p>
<p>Second paragraph.</p>
</section>
</body>
</html>
When looking at the tree-like representation of the document, we see that the section branches into two parts, both of them containing a paragraph with text.
Question not found or loading of the question is still in progress.
Practicing HTML
In this course, we briefly practice using HTML documents in an online editor. This is followed by creating HTML documents locally on our own computers.
The online editor is DartPad, which is used in the FITech 101: Digi & Data course series (currently only in Finnish).
Unless otherwise noted, we are only looking into the HTML documents and HTML code. That is, do not change CSS
tab unless requested otherwise.
Note! When working with the following embedded environment, write only the body of the HTML document to the HTML tab of the editor. That is, if you wish to show the following page.
<!DOCTYPE html>
<html>
<head>
<title>Title</title>
</head>
<body>
<h1>Welcome!</h1>
<p>Now, we will familiarize ourselves with HTML.</p>
</body>
</html>
Only include the following content to the editor.
<h1>Welcome!</h1>
<p>Now, we will familiarize ourselves with HTML.</p>
The first assignments related to HTML are below.
Click the assignment open by clicking the title.
Pressing Run
will show what the document would look like in the browser.